Study: Cutouts vs. Steps Per Frame
Contents
Study: Cutouts vs. Steps Per Frame¶
This is an executable notebook. To open in colab, click the “Launch” icon above (the rocket ship). Once in colab, run the following commands in a new cell to install pytti:
!git clone --recurse-submodules -j8 https://github.com/pytti-tools/pytti-core
%pip install ./pytti-core/vendor/AdaBins
%pip install ./pytti-core/vendor/CLIP
%pip install ./pytti-core/vendor/GMA
%pip install ./pytti-core/vendor/taming-transformers
%pip install ./pytti-core
!python -m pytti.warmup
!touch config/conf/empty.yaml
Specify experiment parameters:¶
cross_product__quality0 = (
("cutouts", (10, 40, 160)),
("steps_per_frame", (20, 80, 160)) # would be way more efficient to just use save_every
)
invariants0 = {
'scenes':'"portrait of a man, oil on canvas"',
'image_model':'VQGAN',
'conf':'empty', # I should just not require conf here...
'seed':12345,
}
# variable imputation doesn't seem to work in the overrides
map_kv = (
('steps_per_frame', ('steps_per_scene','pre_animation_steps', 'display_every', 'save_every')),
)
# this is useful enough that I should just ship it with pytti
from copy import deepcopy
from itertools import (
product,
combinations,
)
from hydra import initialize, compose
from loguru import logger
from pytti.workhorse import _main as render_frames
def build_experiment_parameterizations(
cross_product,
invariants,
map_kv,
):
kargs = []
NAME, VALUE = 0, 1
for param0, param1 in combinations(cross_product, 2):
p0_name, p1_name = param0[NAME], param1[NAME]
for p0_val, p1_val in product(param0[VALUE], param1[VALUE]):
kw = deepcopy(invariants)
kw.update({
p0_name:p0_val,
p1_name:p1_val,
'file_namespace':f"matrix_{p0_name}-{p0_val}_{p1_name}-{p1_val}",
})
# map in "variable imputations"
for k0, krest in map_kv:
for k1 in krest:
kw[k1] = kw[k0]
kargs.append(kw)
kws = [[f"{k}={v}" for k,v in kw.items()] for kw in kargs]
return kargs, kws
def run_experiment_matrix(
kws,
CONFIG_BASE_PATH = "config",
CONFIG_DEFAULTS = "default.yaml",
):
# https://github.com/facebookresearch/hydra/blob/main/examples/jupyter_notebooks/compose_configs_in_notebook.ipynb
# https://omegaconf.readthedocs.io/
# https://hydra.cc/docs/intro/
with initialize(config_path=CONFIG_BASE_PATH):
for k in kws:
logger.debug(k)
cfg = compose(config_name=CONFIG_DEFAULTS,
overrides=k)
render_frames(cfg)
2022-02-24 00:29:16.316 | DEBUG | pytti.warmup:<module>:13 - /home/dmarx/miniconda3/envs/sandbox/lib/python3.9/site-packages/pytti
2022-02-24 00:29:16.317 | DEBUG | pytti.warmup:<module>:27 - /home/dmarx/miniconda3/envs/sandbox/lib/python3.9/site-packages/pytti
2022-02-24 00:29:16.317 | DEBUG | pytti.warmup:ensure_configs_exist:43 - Local config directory detected.
2022-02-24 00:29:17.519 | INFO | pytti.workhorse:<module>:30 - Loading pytti...
2022-02-24 00:29:17.602 | DEBUG | pytti.Notebook:<module>:236 - {'RN50': 'RN50', 'RN101': 'RN101', 'RN50x4': 'RN50x4', 'RN50x16': 'RN50x16', 'RN50x64': 'RN50x64', 'ViTB32': 'ViT-B/32', 'ViTB16': 'ViT-B/16', 'ViTL14': 'ViT-L/14'}
2022-02-24 00:29:19.079 | INFO | pytti.workhorse:<module>:66 - pytti loaded.
2022-02-24 00:29:19.080 | DEBUG | pytti.workhorse:<module>:70 - ['/home/dmarx/proj/pytti-book', '/home/dmarx/miniconda3/envs/sandbox/lib/python39.zip', '/home/dmarx/miniconda3/envs/sandbox/lib/python3.9', '/home/dmarx/miniconda3/envs/sandbox/lib/python3.9/lib-dynload', '', '/home/dmarx/.local/lib/python3.9/site-packages', '/home/dmarx/miniconda3/envs/sandbox/lib/python3.9/site-packages', '/home/dmarx/proj/hypernerf', '/home/dmarx/proj/disco-diffusion/guided-diffusion', '/home/dmarx/proj/disco-diffusion/taming-transformers']
%%capture
kargs, kws = build_experiment_parameterizations(
cross_product__quality0,
invariants0,
map_kv,
)
run_experiment_matrix(kws)
2022-02-24 00:29:19.359 | DEBUG | hydra_plugins.pytti_local_config_searchpath_plugin.searchpath_append_cwd:manipulate_search_path:18 - /home/dmarx/proj/pytti-book/config/
2022-02-24 00:29:19.360 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=10', 'steps_per_frame=20', 'file_namespace=matrix_cutouts-10_steps_per_frame-20', 'steps_per_scene=20', 'pre_animation_steps=20', 'display_every=20', 'save_every=20']
2022-02-24 00:29:19.436 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:19.437 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:19.543 | DEBUG | pytti.Notebook:load_clip:264 - Loading CLIP...
2022-02-24 00:29:24.398 | DEBUG | pytti.Notebook:load_clip:266 - CLIP loaded.
2022-02-24 00:29:24.399 | DEBUG | pytti.workhorse:do_run:242 - 10
2022-02-24 00:29:24.402 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:29:24.803 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:29:24.804 | INFO | pytti.Image.VQGANImage:init_vqgan:230 - /home/dmarx/.cache/vqgan
2022-02-24 00:29:24.805 | DEBUG | pytti.Image.VQGANImage:init_vqgan:234 - /home/dmarx/.cache/vqgan/sflckr.yaml
2022-02-24 00:29:24.805 | DEBUG | pytti.Image.VQGANImage:init_vqgan:235 - /home/dmarx/.cache/vqgan/sflckr.ckpt
2022-02-24 00:29:29.804 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-20/matrix_cutouts-10_steps_per_frame-20(4)_settings.txt
2022-02-24 00:29:29.810 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:29:29.824 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:29:34.411 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-20/matrix_cutouts-10_steps_per_frame-20(4)_1.png
2022-02-24 00:29:34.798 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:29:35.028 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=10', 'steps_per_frame=80', 'file_namespace=matrix_cutouts-10_steps_per_frame-80', 'steps_per_scene=80', 'pre_animation_steps=80', 'display_every=80', 'save_every=80']
2022-02-24 00:29:35.085 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:35.086 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:35.210 | DEBUG | pytti.workhorse:do_run:242 - 10
2022-02-24 00:29:35.213 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:29:35.225 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:29:35.227 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-80/matrix_cutouts-10_steps_per_frame-80(3)_settings.txt
2022-02-24 00:29:35.231 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:29:35.242 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:29:53.133 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-80/matrix_cutouts-10_steps_per_frame-80(3)_1.png
2022-02-24 00:29:53.508 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:29:53.757 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=10', 'steps_per_frame=160', 'file_namespace=matrix_cutouts-10_steps_per_frame-160', 'steps_per_scene=160', 'pre_animation_steps=160', 'display_every=160', 'save_every=160']
2022-02-24 00:29:53.809 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:53.810 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 10, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-10_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:29:53.908 | DEBUG | pytti.workhorse:do_run:242 - 10
2022-02-24 00:29:53.911 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:29:53.922 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:29:53.925 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-160/matrix_cutouts-10_steps_per_frame-160(3)_settings.txt
2022-02-24 00:29:53.929 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:29:53.939 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:30:29.485 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-10_steps_per_frame-160/matrix_cutouts-10_steps_per_frame-160(3)_1.png
2022-02-24 00:30:29.865 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:30:30.114 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=40', 'steps_per_frame=20', 'file_namespace=matrix_cutouts-40_steps_per_frame-20', 'steps_per_scene=20', 'pre_animation_steps=20', 'display_every=20', 'save_every=20']
2022-02-24 00:30:30.167 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:30.168 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:30.264 | DEBUG | pytti.workhorse:do_run:242 - 40
2022-02-24 00:30:30.266 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:30:30.278 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:30:30.281 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-20/matrix_cutouts-40_steps_per_frame-20(3)_settings.txt
2022-02-24 00:30:30.285 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:30:30.295 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:30:35.487 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-20/matrix_cutouts-40_steps_per_frame-20(3)_1.png
2022-02-24 00:30:35.911 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:30:36.149 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=40', 'steps_per_frame=80', 'file_namespace=matrix_cutouts-40_steps_per_frame-80', 'steps_per_scene=80', 'pre_animation_steps=80', 'display_every=80', 'save_every=80']
2022-02-24 00:30:36.204 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:36.205 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:36.305 | DEBUG | pytti.workhorse:do_run:242 - 40
2022-02-24 00:30:36.307 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:30:36.319 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:30:36.322 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-80/matrix_cutouts-40_steps_per_frame-80(3)_settings.txt
2022-02-24 00:30:36.327 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:30:36.337 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:30:57.724 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-80/matrix_cutouts-40_steps_per_frame-80(3)_1.png
2022-02-24 00:30:58.153 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:30:58.391 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=40', 'steps_per_frame=160', 'file_namespace=matrix_cutouts-40_steps_per_frame-160', 'steps_per_scene=160', 'pre_animation_steps=160', 'display_every=160', 'save_every=160']
2022-02-24 00:30:58.445 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:58.446 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 40, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-40_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:30:58.546 | DEBUG | pytti.workhorse:do_run:242 - 40
2022-02-24 00:30:58.549 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:30:58.561 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:30:58.563 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-160/matrix_cutouts-40_steps_per_frame-160(3)_settings.txt
2022-02-24 00:30:58.567 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:30:58.578 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:31:41.069 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-40_steps_per_frame-160/matrix_cutouts-40_steps_per_frame-160(3)_1.png
2022-02-24 00:31:41.488 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:31:41.727 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=160', 'steps_per_frame=20', 'file_namespace=matrix_cutouts-160_steps_per_frame-20', 'steps_per_scene=20', 'pre_animation_steps=20', 'display_every=20', 'save_every=20']
2022-02-24 00:31:41.779 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:31:41.781 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 20, 'steps_per_frame': 20, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 20, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-20', 'allow_overwrite': False, 'display_every': 20, 'clear_every': 0, 'display_scale': 1, 'save_every': 20, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:31:41.877 | DEBUG | pytti.workhorse:do_run:242 - 160
2022-02-24 00:31:41.879 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:31:41.891 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:31:41.895 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-20/matrix_cutouts-160_steps_per_frame-20(3)_settings.txt
2022-02-24 00:31:41.899 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:31:41.909 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:31:50.299 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-20/matrix_cutouts-160_steps_per_frame-20(3)_1.png
2022-02-24 00:31:50.877 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:31:51.238 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=160', 'steps_per_frame=80', 'file_namespace=matrix_cutouts-160_steps_per_frame-80', 'steps_per_scene=80', 'pre_animation_steps=80', 'display_every=80', 'save_every=80']
2022-02-24 00:31:51.290 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:31:51.291 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 80, 'steps_per_frame': 80, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 80, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-80', 'allow_overwrite': False, 'display_every': 80, 'clear_every': 0, 'display_scale': 1, 'save_every': 80, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:31:51.387 | DEBUG | pytti.workhorse:do_run:242 - 160
2022-02-24 00:31:51.389 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:31:51.401 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:31:51.406 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-80/matrix_cutouts-160_steps_per_frame-80(3)_settings.txt
2022-02-24 00:31:51.409 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:31:51.419 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:32:26.332 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-80/matrix_cutouts-160_steps_per_frame-80(3)_1.png
2022-02-24 00:32:26.923 | INFO | pytti.workhorse:_main:587 - Complete.
2022-02-24 00:32:27.289 | DEBUG | __main__:run_experiment_matrix:47 - ['scenes="portrait of a man, oil on canvas"', 'image_model=VQGAN', 'conf=empty', 'seed=12345', 'cutouts=160', 'steps_per_frame=160', 'file_namespace=matrix_cutouts-160_steps_per_frame-160', 'steps_per_scene=160', 'pre_animation_steps=160', 'display_every=160', 'save_every=160']
2022-02-24 00:32:27.342 | DEBUG | pytti.workhorse:_main:187 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '${user_cache:}', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:32:27.343 | DEBUG | pytti.workhorse:_main:188 - {'scenes': 'portrait of a man, oil on canvas', 'scene_prefix': '', 'scene_suffix': '', 'direct_image_prompts': '', 'init_image': '', 'direct_init_weight': '', 'semantic_init_weight': '', 'image_model': 'VQGAN', 'vqgan_model': 'sflckr', 'animation_mode': '3D', 'width': 180, 'height': 112, 'steps_per_scene': 160, 'steps_per_frame': 160, 'interpolation_steps': 0, 'learning_rate': None, 'reset_lr_each_frame': True, 'seed': 12345, 'cutouts': 160, 'cut_pow': 2, 'cutout_border': 0.25, 'border_mode': 'clamp', 'field_of_view': 60, 'near_plane': 1, 'far_plane': 10000, 'translate_x': '-1700*sin(radians(1.5))', 'translate_y': '0', 'translate_z_3d': '(50+10*t)*sin(t/10*pi)**2', 'rotate_3d': '[cos(radians(1.5)), 0, -sin(radians(1.5))/sqrt(2), sin(radians(1.5))/sqrt(2)]', 'rotate_2d': '5', 'zoom_x_2d': '0', 'zoom_y_2d': '0', 'sampling_mode': 'bicubic', 'infill_mode': 'wrap', 'pre_animation_steps': 160, 'lock_camera': True, 'pixel_size': 4, 'smoothing_weight': 0.02, 'random_initial_palette': False, 'palette_size': 6, 'palettes': 9, 'gamma': 1, 'hdr_weight': 0.01, 'palette_normalization_weight': 0.2, 'show_palette': False, 'target_palette': '', 'lock_palette': False, 'frames_per_second': 12, 'direct_stabilization_weight': '', 'semantic_stabilization_weight': '', 'depth_stabilization_weight': '', 'edge_stabilization_weight': '', 'flow_stabilization_weight': '', 'video_path': '', 'frame_stride': 1, 'reencode_each_frame': True, 'flow_long_term_samples': 1, 'ViTB32': True, 'ViTB16': False, 'RN50': False, 'RN50x4': False, 'file_namespace': 'matrix_cutouts-160_steps_per_frame-160', 'allow_overwrite': False, 'display_every': 160, 'clear_every': 0, 'display_scale': 1, 'save_every': 160, 'backups': 5, 'show_graphs': False, 'approximate_vram_usage': False, 'models_parent_dir': '/home/dmarx/.cache', 'gradient_accumulation_steps': 1, 'conf': {}}
2022-02-24 00:32:27.441 | DEBUG | pytti.workhorse:do_run:242 - 160
2022-02-24 00:32:27.443 | INFO | pytti.workhorse:parse_scenes:110 - Loading prompts...
2022-02-24 00:32:27.455 | INFO | pytti.workhorse:parse_scenes:120 - Prompts loaded.
2022-02-24 00:32:27.458 | INFO | pytti.workhorse:do_run:520 - Settings saved to /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-160/matrix_cutouts-160_steps_per_frame-160(3)_settings.txt
2022-02-24 00:32:27.462 | INFO | pytti.workhorse:do_run:533 - Running prompt:
2022-02-24 00:32:27.473 | DEBUG | pytti.ImageGuide:report_out:364 - Step 0 losses:
2022-02-24 00:33:37.261 | DEBUG | pytti.ImageGuide:save_out:416 - /home/dmarx/proj/pytti-book/images_out//matrix_cutouts-160_steps_per_frame-160/matrix_cutouts-160_steps_per_frame-160(3)_1.png
2022-02-24 00:33:37.869 | INFO | pytti.workhorse:_main:587 - Complete.
# https://pytorch.org/vision/master/auto_examples/plot_visualization_utils.html#visualizing-a-grid-of-images
# sphinx_gallery_thumbnail_path = "../../gallery/assets/visualization_utils_thumbnail2.png"
from pathlib import Path
import numpy as np
import matplotlib.pyplot as plt
from torchvision.io import read_image
import torchvision.transforms.functional as F
from torchvision.utils import make_grid
plt.rcParams["savefig.bbox"] = 'tight'
plt.rcParams['figure.figsize'] = 20,20
def show(imgs):
if not isinstance(imgs, list):
imgs = [imgs]
fix, axs = plt.subplots(ncols=len(imgs), squeeze=False)
for i, img in enumerate(imgs):
img = img.detach()
img = F.to_pil_image(img)
axs[0, i].imshow(np.asarray(img))
axs[0, i].set(xticklabels=[], yticklabels=[], xticks=[], yticks=[])
return fix, axs
images = []
for k in kargs:
fpath = Path("images_out") / k['file_namespace'] / f"{k['file_namespace']}_1.png"
images.append(read_image(str(fpath)))
nr = len(cross_product__quality0[0][-1])
grid = make_grid(images, nrow=nr)
fix, axs = show(grid)
ax0_name, ax1_name = cross_product__quality0[0][0], cross_product__quality0[1][0]
fix.savefig(f"TestMatrix_{ax0_name}_{ax1_name}.png")
# to do:
# * label axes and values
# * track and report runtimes for each experiment
# * track and report runtime of notebook
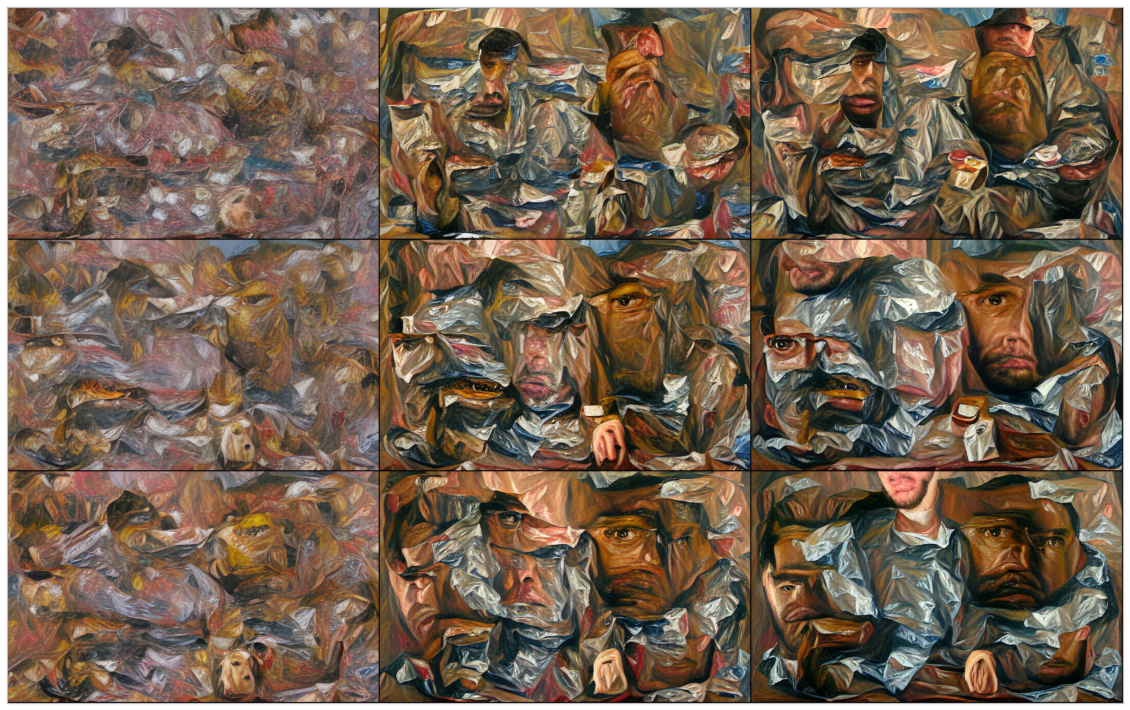